During the construction of the accessibility tree, for certain elements the browser computes a name, the accessible name.
Notice in the example below how Chrome's accessibility tree lists a string after the role of the object. This string represents the accessible name.
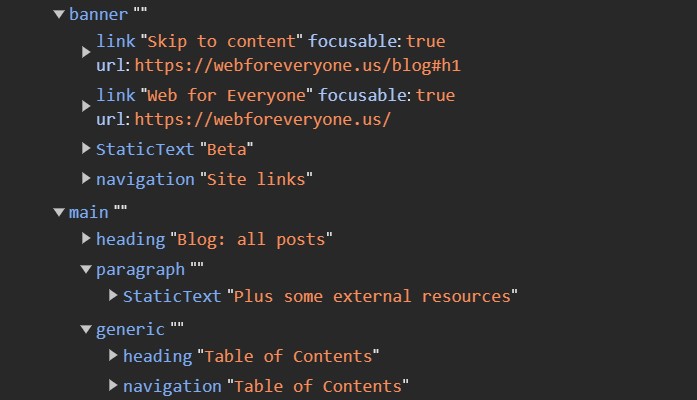
An empty string indicates that the given element does not have a name. Is that a bad thing? Not necessarily. Not every element needs to have a name. We can actually group the tree objects into "must-have", "could-have", and "must-not-have" a name categories. In this post, we will look at examples of elements from each category, and for those that do get a name, how the browser computes it.
Must-have-a-name elements
These are interactive elements for which the name is required (not an exhaustive list):
links and buttons
all form elements, like input fields and checkboxes
headings
When a screen reader user tabs to an input
field, the name of the element is announced. This is how the user knows what input the field expects: an email, a name, or a credit card number, to give a few examples. If a voice user wants to activate a button, the button needs to have a name in order for the user to activate it with the "Click name-of-button" command.
Notice in the screenshot from the Chromium accessibility tree that the headings also have a name. You wouldn't typically think of a heading as an interactive element, but it is interactive for screen reader users. They have the option to navigate the page by headings by pressing the H key , and the name of the heading, as well as its level (a number from 1 to 6), is announced when they reach it.
Did you know? about landmarks
The WebAIM's Screen Reader User Survey shows that navigating by headings is the preferred way to move through a page for many screen reader users.
A little over 70% of the respondents of the 2024 survey chose "navigate headings" as their preferred method.
The computation algorithm
To create the accessible name, the browser follows the rules set by the accessible name and description computation algorithm. The algorithm takes into account the visible text or label of the element, but several other attributes are considered as well.
1. The "aria-labelledby" attribute
It has the highest priority among all possible attributes, including the visible text. It references the ID of another element that has text, either visual or hidden. The text of that element then becomes the name of the current element.
<h2 id="intro-to-accessibility">Intro to Accessibility<h2>
<section aria-labelledby="intro-to-accessibility">
// content of the region
</section>
In the example above, we created a region on the page. Think of regions as the broad sections of a webpage that contain similar content. The header, footer, or main content area are examples of regions, also called landmarks, built from their corresponding HTML tags. If you want to create your own custom region, you can use a section
tag but you must give it a name with the aria-labelledby
or aria-label
attribute. In the accessibility tree, the element maps to an object with role region
. Without assigning it a name you simply have a section element that maps to a generic
role, so nothing more than a div-like container.
Please note that regions or landmarks help screen reader users orient themselves on the page. Apart from using the expected native landmarks - header, navigation, main, footer - crowding the page with too many custom regions kind of defeats their purpose. Don't forget that we also have the aside
tag which maps to a landmark with role complementary
. For a chunk of content that is related to the main content, but not essential to or part of it, this landmark might be what what you need instead of a custom region.
2. The "aria-label" attribute
It takes a string value that is not visible on the page, and it's only announced to the screen reader user. It's the second in line in terms of priority, so without an aria-labelledby
present it will be used to compute the accessible name. In the example below, the link to a LinkedIn profile has no visible text, and the icon is hidden with aria-hidden
. When computing the accessible name for the link, the browser will use the value of the aria-label
attribute.
<a aria-label="LinkedIn" href="https://www.linkedin.com/in/yourname/">
<i className="fa-brands fa-linkedin" aria-hidden="true"></i>
</a>
One problem with a name created this way is that there's no guarantee it will to be auto-translated. This unfortunately prevents your content from being accessible to a global audience, a process called "internationalization". Screen reader or voice users who speak a different language than the one in which the page was written could use a tool like Google Translate to read the content, but the accessible names computed from the aria-label
attribute might not be translated. In this scenario, they will have a hard time understanding the purpose of or interacting with those elements. For this reason, even if it's the second most powerful attribute, it's best to consider one of the other - better - attributes first.
A second problem is specific to any approach that doesn't rely on visible text. Voice users need to know the name of the element in order to activate it, so it's your responsibility to make sure the name is obvious from context or that the icon is recognizable, like in our example.
3. Visible text or label
Adding text between the opening and closing tags or attaching a label to a form field will provide the name for the element. It is the best choice for all users. The browser will use it to compute the accessible name and it will be announced to the screen reader user. There will be no issues with internationalization, and the voice user can activate the element by saying its name, without having to guess it.
4. Text defined by a visually-hidden class
This is a CSS class that hides text visually, but makes it available to screen readers. In order to implement the CSS class correctly, use white-space: nowrap
, otherwise the spaces between words might be ignored and screen readers could announce the text as a single continuous string.
A code example of a visually-hidden class is provided in the CodePen below. If you cannot have visible text and need to use an icon or image, this approach is better than using an aria-label
attribute since there will be no issues with internationalization.
5. The "alt" attribute of an image
You can use it with interactive images. With buttons or links that contain an image and no visible text or ARIA attributes, the browser will take the alt
attribute to compute the accessible name.
Note that this is slightly more verbose. So, a button wrapping an image of x can have an alt="Close"
and it will be announced as "Close graphic, button".
<button>
<img alt="Close" src="x-mark.svg"/>
</button>
Remember: An img
element is announced as either graphic or image, depending on the screen reader.
6. The "title" attribute
This is an attribute that can be added to the opening HTML tag of an element. Technically, it can be used to compute the accessible name, but it has poor browser and screen reader support, so it's best to avoid it.
Note: Do not confuse this attribute with the <title>
element, which is added to the head
of the html document to provide a title for the webpage.
To summarize:
✔ If there's no visible text or label, or at least text with a visually-hidden
class, the algorithm relies on the content of certain HTML and ARIA attributes: - aria-labelledby
, aria-label
, alt
.
✔ The ARIA attributes are given priority even when the element has visible text or a label. The idea being that they were added with a purpose: to offer a more descriptive or tailored name for the element. So, if the element has descriptive visible text or label, there is no need do add an aria-labelledby
or aria-label
.
Could-have-a-name elements
Examples of elements that belong to this category are those with a role of landmark. For instance, when using the nav
tag, we create an element that maps to an object with role navigation
in the accessibility tree. The screen readers will announce it as "navigation landmark". It does not require a name since users recognize it by its role of "navigation".
However, if we add a second navigation landmark on the page, then we must assign to each a unique name so that a screen reader user could tell them apart. We could use eitheraria-labelledby
or aria-label
. Please note that some landmarks should not be used more than once on a page. You should always have one, but just one, main
element.
The code below creates a navigation landmark for a table of contents.
<h2 id="table-of-contents">Table of Contents<h2>
<nav aria-labelledby="table-of-contents">
// table of contents links
</nav>
Did you know? about landmarks
Screen reader users have a way to quickly jump between sections of a page. Depending on the screen reader, pressing the D or the R key allows them to navigate between landmarks.
Must-not-have-a-name elements
The ARIA specification uses the term "prohibited" when referring to elements that should not have a name. Take for example a div
or a span
that have a default role of generic
. This role has no semantic meaning, so the element should remain a "nameless container".
There's absolutely no need to use an aria-label
or aria-labelledby
attribute on these elements. Unless, of course, you change their role to something that requires a name, in which case you'll refer to the "must-have" category above.
What next?
We learned about the role the accessibility tree plays in making the web accessible, and how the browser computes the accessible name for different elements. We can now have a look at the most common accessibility issues, but first, a few key takeaways:
The accessibility tree represents the structure of a webpage in a way that is meaningful for users of assistive technologies.
Certain objects in the accessibility tree must have a name, and it has to be descriptive in order to convey their purpose.
Semantic HTML tags should always be your first choice. They have built-in roles and properties that make your content accessible.
Read next: The most common acessibility bugs